Task 4: GUI¶
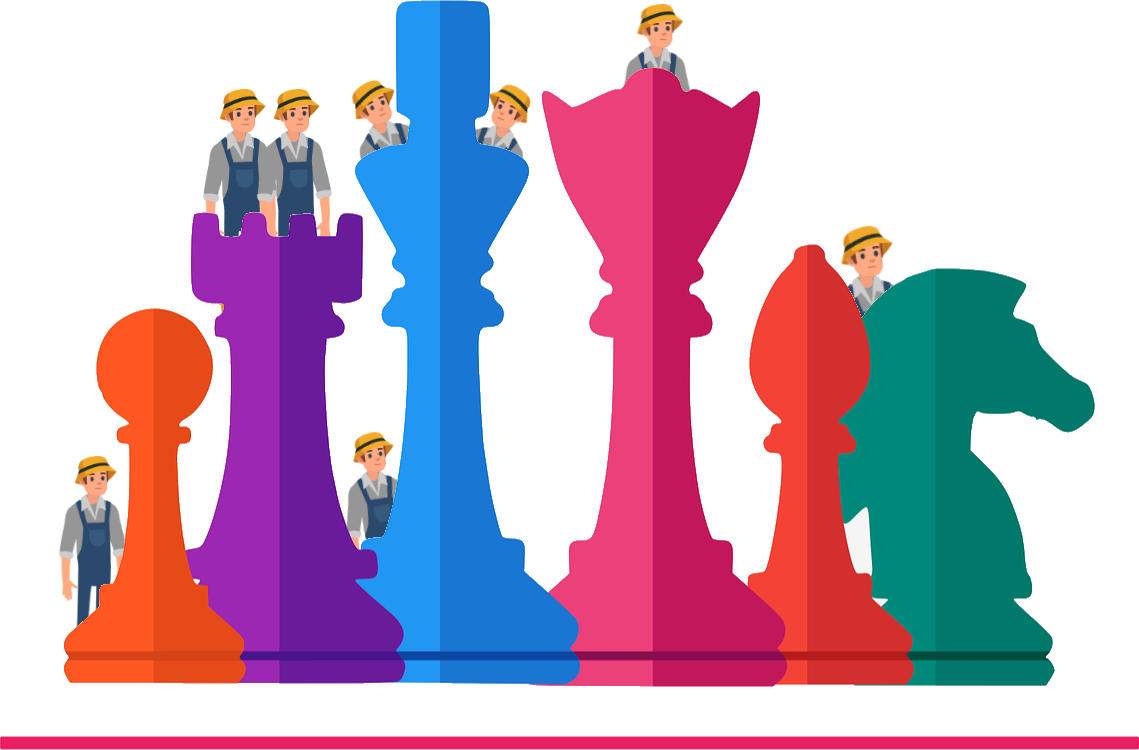
Your task is to implement a graphical user interface (GUI) for a hot-seat game of pawn chess (“Bauernschach”). The GUI should be based on JavaFX and implement separation of concerns through the Model-View-Controller (MVC) pattern.
The minimum requirement for passing the functional grading of this task: It should be possible to start a new game and make a forward-move with any pawn. The executed move should be visible, but it is not necessary to show the possible moves after selecting a pawn.
We recommend to first implement basic behavior, and then add advanced requirements later.
Use the given template. You can modify all given code, if necessary. The GUI should be fully responsive at all times.
Pawn Chess Rules¶
Pawn Chess is played on a traditional chess board of 8x8 tiles. Rows are identified with letters A-H, columns are identified with numbers 1-8.
Two players play against each other: There’s “White” and “Black”.
Game setup¶
Player White starts with 8 pawns on row A (columns 1-8), player Black starts with 8 pawns on row H (columns 1-8).
Winning condition¶
Whoever is able to reach the opponent’s start row with a pawn first wins the game.
If a pawn of player White moves onto any field in row H, player White wins.
If a pawn of player Black moves onto any field in row A, player Black wins.
If a player has no pawns left, the game ends early and the opposing player wins.
Game play¶
Player White starts the game.
The pawns of player White start at row A and move towards row H. THe pawns of player Black start at row H and move towards row A.
The players take turns until the game ends. In each turn, the current player either moves one pawn, or passes.
Each pawn can be moved according to the following two rules:
If the pawn is on its start row and both the field in front and the field two in front of the pawn is free, the pawn can move forward two fields, onto that field.
If the field in front of the pawn is free, the pawn can move forward one field.
If there’s an opposing pawn on a field one forward and one left or right to the pawn (diagonal), the pawn can move onto the field and the opposing pawn is removed from the game.
Otherwise, the pawn can not move in that turn.
If no pawn can move, the player has to pass and it’s the other player’s turn.
Hot-seat Game¶
We play pawn chess as a hot-seat multiplayer game: Two players play against each other on a single computer, and use the same mouse for playing.
GUI¶
Implement three screens: A welcome screen, a gameplay screen, and a victory screen. The game has the following flow between screens: Welcome screen -> Gameplay screen -> Victory screen -> Gameplay screen -> …
Welcome screen¶
The welcome screen consists of the following components:
A text banner “Bauernschach”
A button “Start game”. When the button is clicked, the welcome screen disappears, a new pawn-chess game starts, and the game screen is displayed.
Example look:
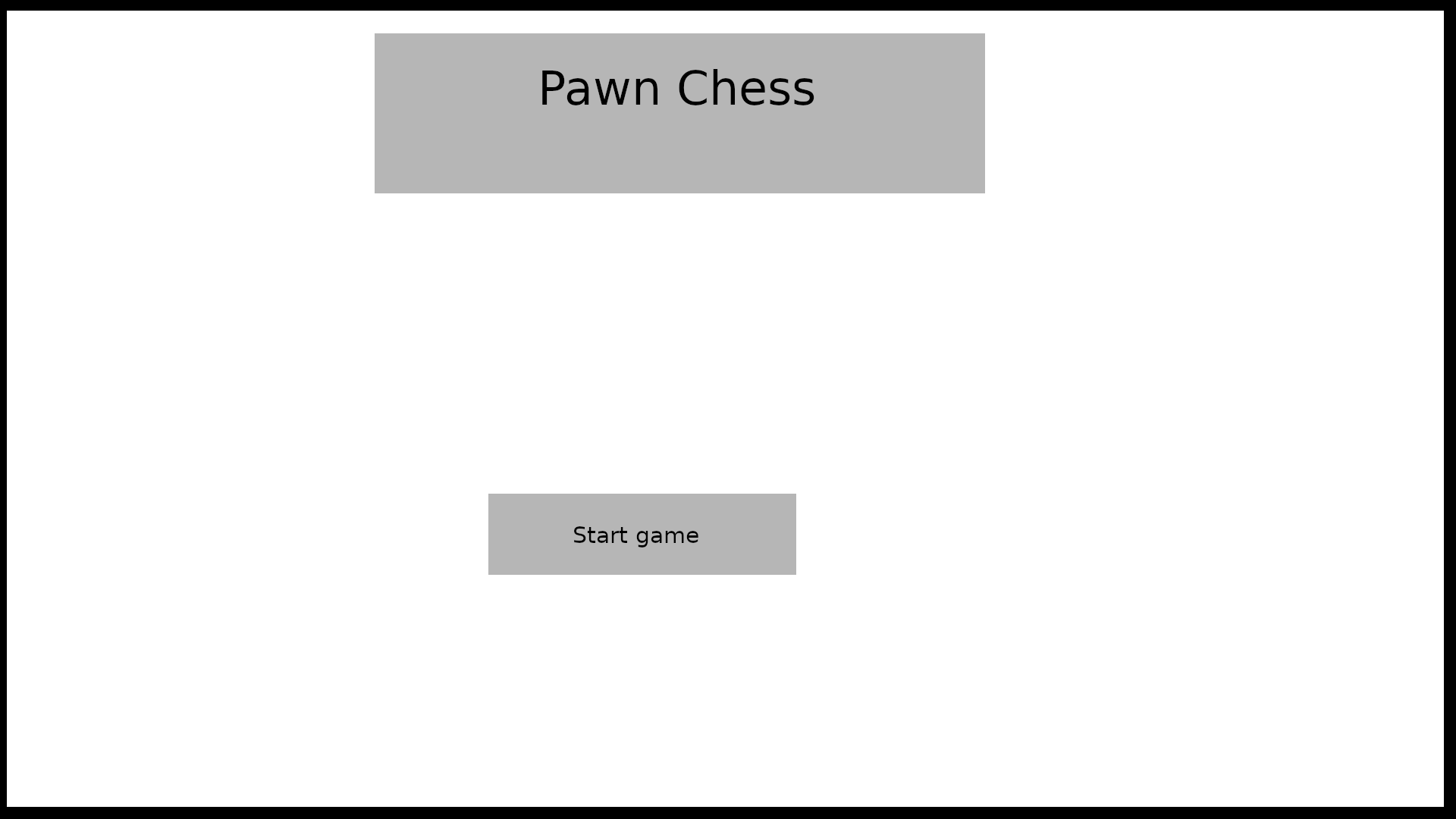
Gameplay screen¶
The gameplay screen consists of two panels.
The left panel displays the current game board, with the player whose turn it is currently at the bottom of the board (that means the board rotates 180 degrees after each turn).
The left panel shows the following behavior:
Users can click on any of the pawns of the current player to select that pawn. This highlights the pawn.
Advanced requirement: This also highlights all possible tiles the pawn can move onto.
After selecting a pawn, users can click on any of the valid tiles to move the pawn onto that tile.
Advanced requirement: The pawn does not just disappear at its current tile and reappear at the new tile, but the move is animated with a smooth transition from one tile to the other.
After moving a pawn, the next player’s turn starts. Advaned requirement: The board turns by 180 degrees to reflect that the next player’s turn starts. The current player is always at the bottom of the displayed board.
The right panel displays the following components:
A text banner “Pawn Chess”
A text “Current player: …” that displays the current player
Example look:
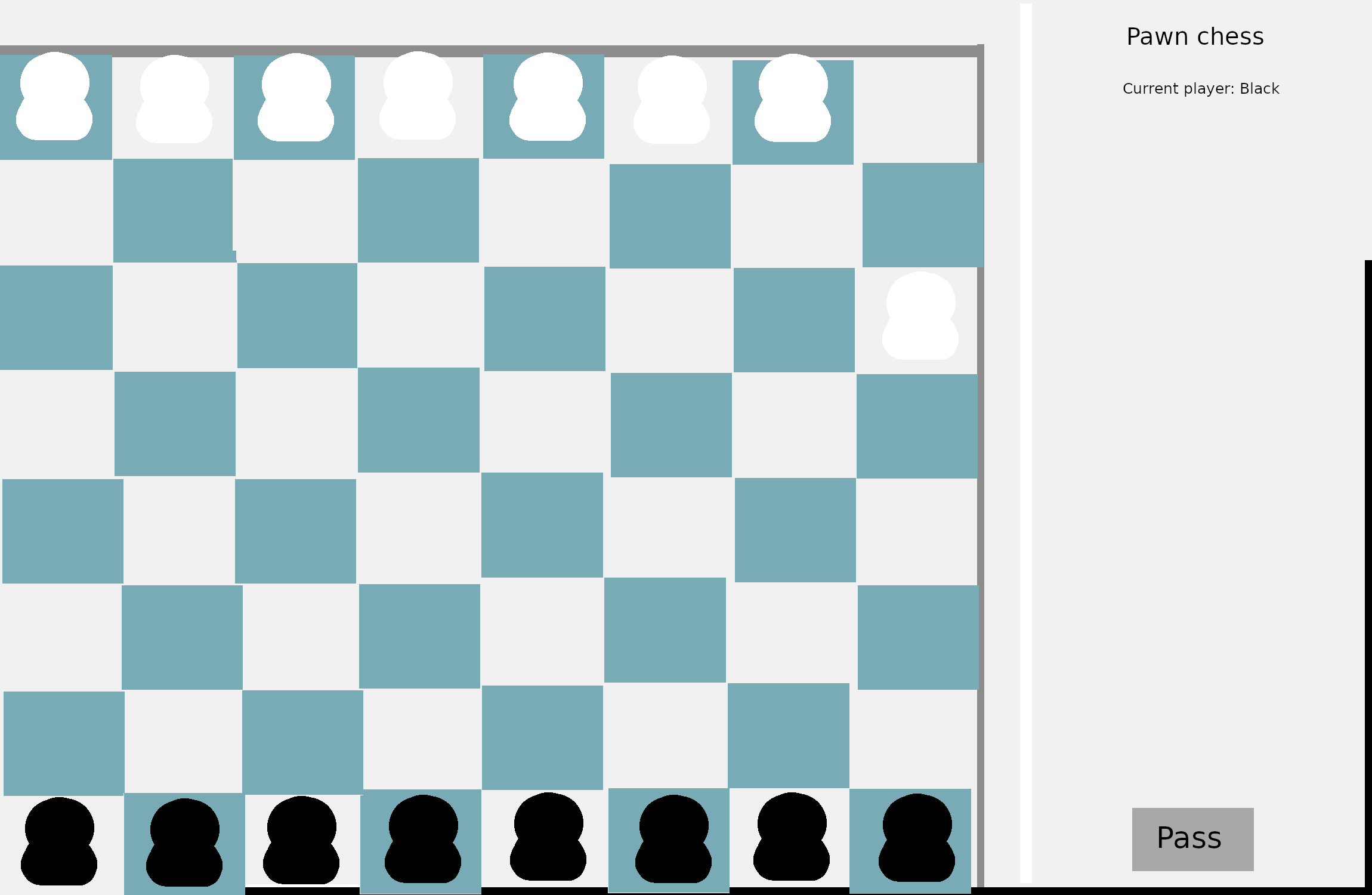
Rotated for other player:
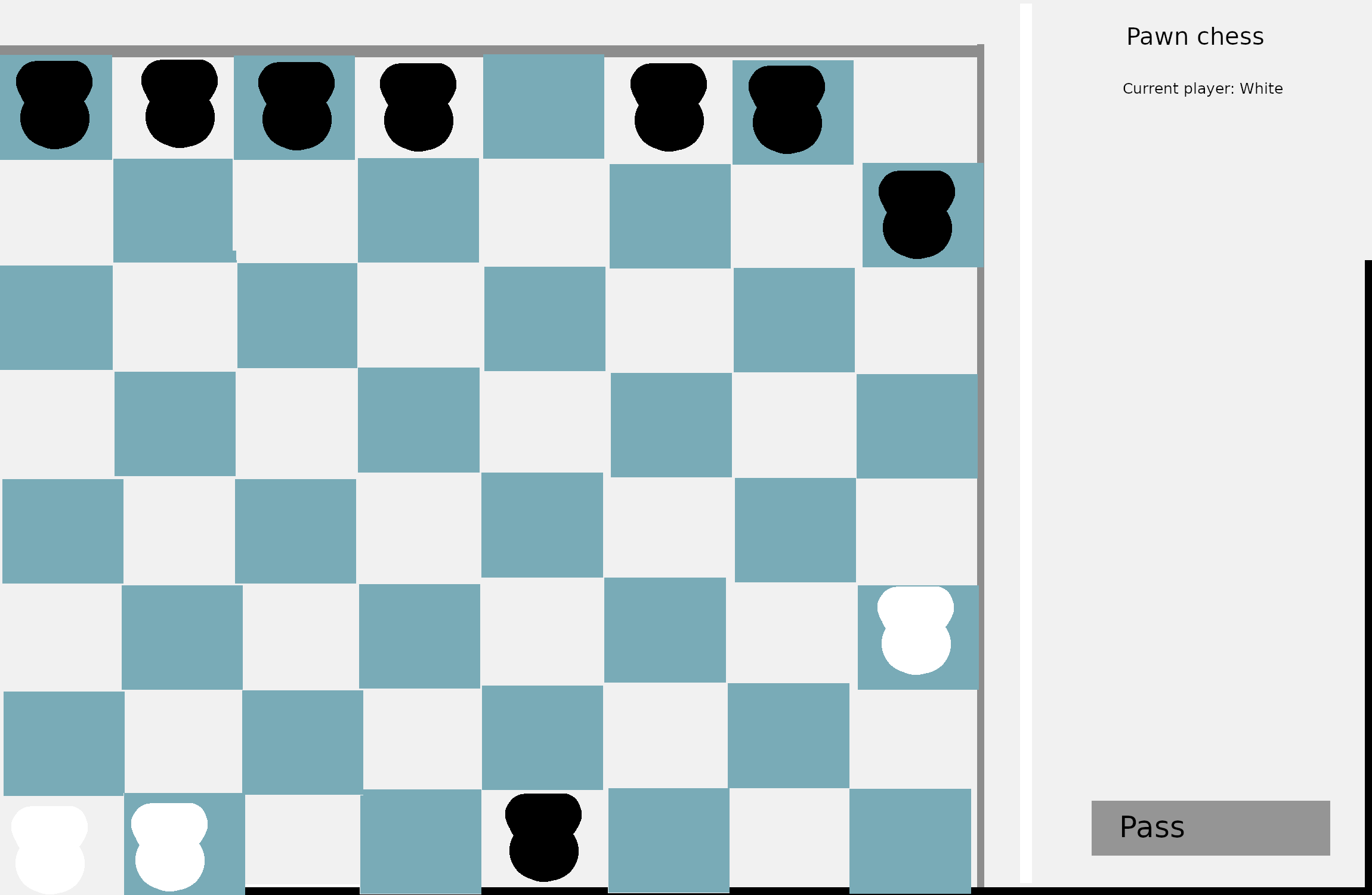
Victory screen¶
If the game is over, a victory screen appears. The screen can be shown above the game screen, or replace it.
The victory screen consists of the following components:
A text banner shows the winner (or draw, if there is no winner).
A button “Start new game”. When clicked, the victory screen disappears, a new game is started, and the game screen is shown again.
Example look:
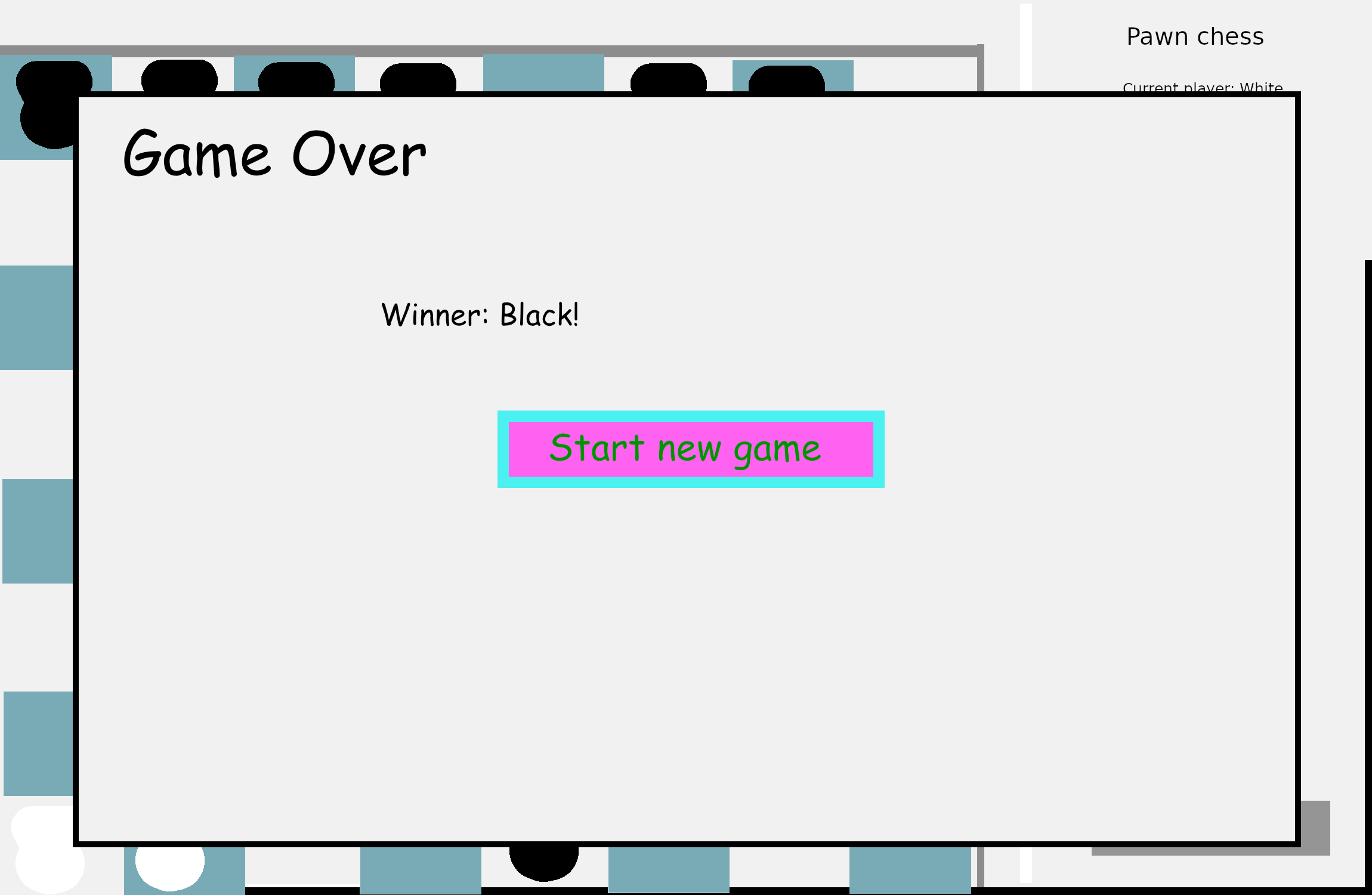
The styling and positioning of components, unless stated in the text, can be arbitrary.
General Requirements¶
Documentation of the source code is part of the exercise and will be graded. The Google Java Style Guide must be fulfilled.
Your solution must be anonymous. Your name may not appear in the solution.
Your solution may not contain compiled data (no
build
directory or.class
files). Please check the content of your ZIP file before uploading.
Helpful Resources¶
Some helpful resources:
JavaFX JavaDoc for detailed references
Good luck!